将一个复杂对象的构建与它的表示分离,使得同样的构建过程可以创建不同的表示。
1 2 3 4 5
| Man man = new Man.Builder("cbry" , "Smart Brain" , "Nimble Hand") .setBook("effective java") .setPhone("MEIZU") .setComputer("Dell") .build();
|
意图
将一个复杂对象的构建与它的表示分离,使得同样的构建过程可以创建不同的表示。
适用性
当创建复杂对象的算法应该独立于该对象的组成部分以及它们的装配方式时。
当构造过程必须允许被构造对象有不同的表示时。
UML
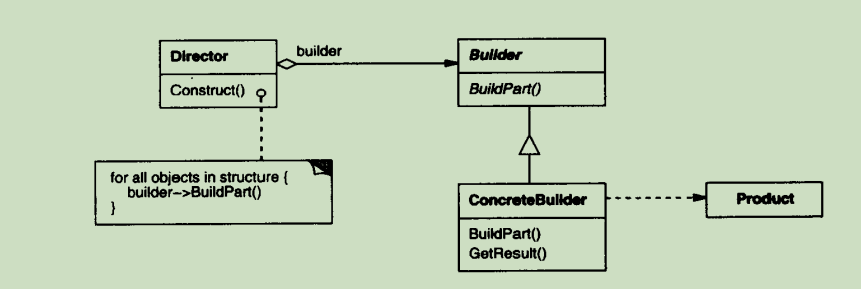
效果
它使你可以改变一个产品的内部表示
它将构造代码和表示代码分开
它使你可对构造过程进行更精细的控制
链式调用Builder模式
我们更常用的起始是原始Builder模式的链式调用Builder模式。比如gson使用中的创建一个Gson对象:
1 2 3 4
| Gson gson = new GsonBuilder() .setDateFormat("yyyy-MM-dd HH:mm:ss") .serializeNulls() .create();
|
链式调用Builder实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69
| package com.builder;
public class Man { private final String name; private final String head; private final String hand; private String computer; private String phone; private String book;
public Man(Builder builder){ this.name = builder.name; this.head = builder.head; this.hand = builder.hand; }
public static class Builder{ private String name; private String head; private String hand; private String computer; private String phone; private String book;
public Builder(String name , String head , String hand){ this.name = name; this.head = head; this.hand = hand; }
public Builder setComputer(String computer) { this.computer = computer; return this; }
public Builder setPhone(String phone) { this.phone = phone; return this; }
public Builder setBook(String book) { this.book = book; return this; }
public Man build(){ return new Man(this); }
}
public static void main(String[] args) { Man man = new Man.Builder("cbry" , "Smart Brain" , "Nimble Hand") .setBook("effective java") .setPhone("MEIZU") .setComputer("Dell") .build(); } }
|